- Autocomplete - show possible functions and parameters
- Syntax highlight - highlight the syntax of the formula
- Error detection - detect errors in the formula and errors
- Point & Compose - eg. when you open a parenthesis you go into a mode where you can click a cell to compose it into the formula, or you can use the keyboard to pick a cell.
- Positioning - It can be displayed on a fixed bar on top or as a floating element that when the spreadsheet is scrolled out of position it should show a label with the cell name.
=SUM(A1:B1)
would be represented as:
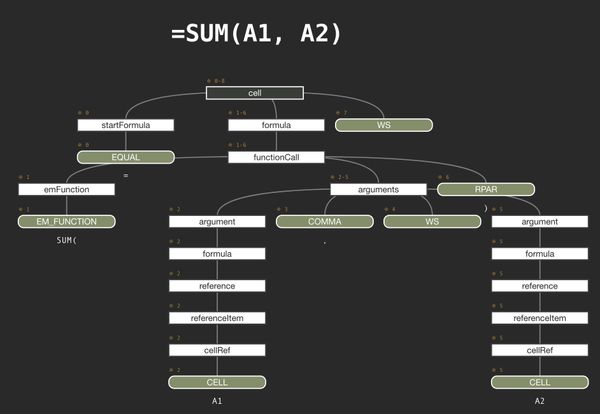
Here's a simple example of how to use the library:
final machine = StateMachine.create(
(g) => g
..initial<Alive>()
..state<Alive>(
builder: (b) => b
..initial<Young>()
..on<OnBirthday, Young>(...)
..on<OnBirthday, MiddleAged>(...)
..on<OnBirthday, Old>(...)
..on<OnDeath, Purgatory>()
..state<Young>()
..state<MiddleAged>()
..state<Old>(),
)
..state<Dead>(),
);